GitOps for Feature Flags Using Terraform and Terrateam
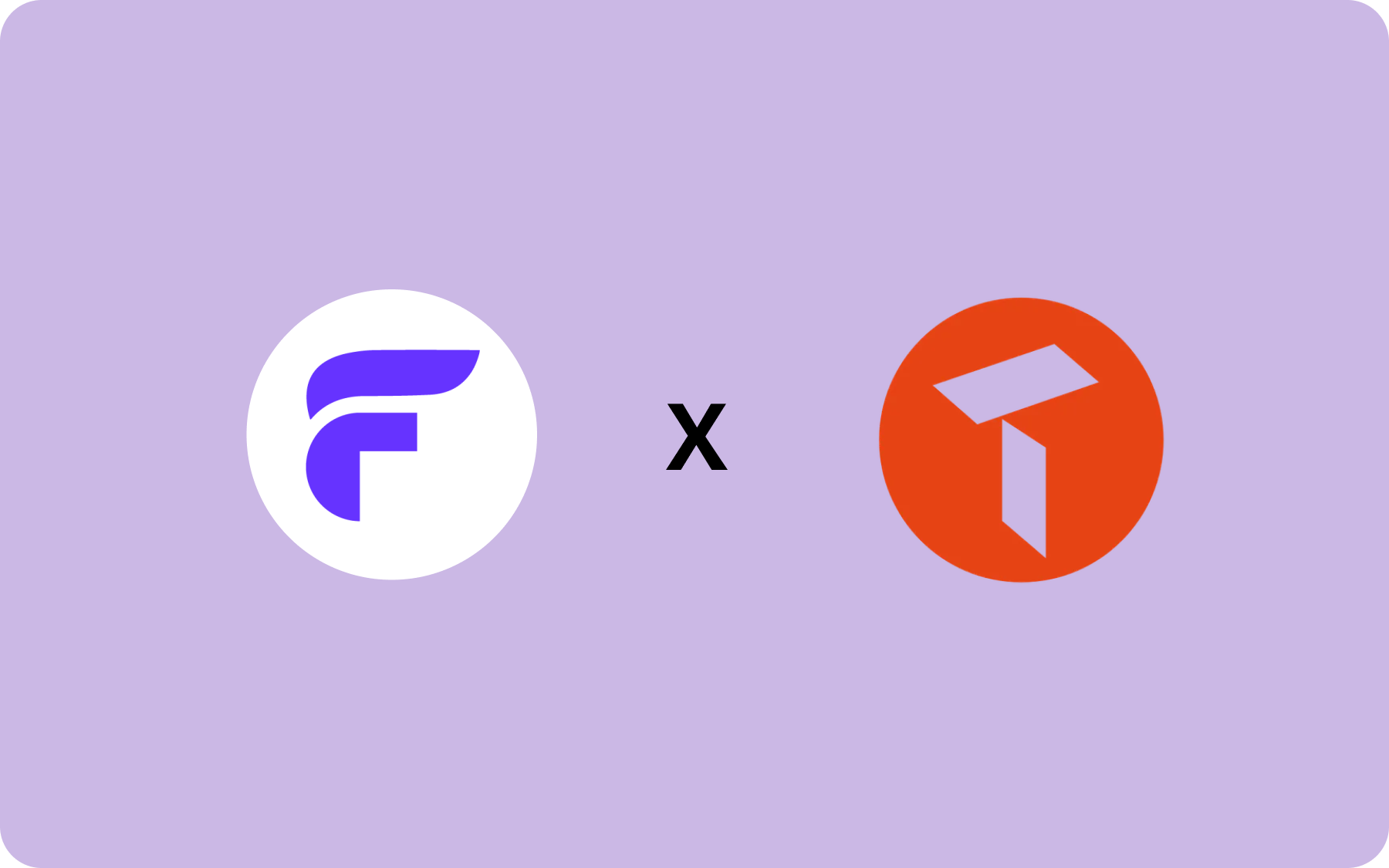
For teams using GitOps, feature flags can fit naturally into existing workflows. Whether you're rolling out new features or reverting changes in production, managing Flagsmith flags through GitOps with Terrateam keeps updates transparent, tested, and aligned with your codebase.
Why choose a GitOps approach for feature flags?
Managing feature flags with GitOps treats them like any other part of your infrastructure. The codebase becomes the single source of truth. Every change is tracked, reviewed, and deployed through the same workflows developers already use. If something goes wrong, rolling back is as simple as reverting a PR.
Using Terraform with Flagsmith makes feature flags declarative and version-controlled. Updates go through pull request reviews and CI/CD pipelines, ensuring consistency across environments. When a PR is merged, the new flag configuration is applied automatically.
This approach keeps teams aligned. Developers, QA, and product managers can see and review flag changes alongside other code updates. Everything is visible, tested, and versioned, reducing surprises and making feature releases more predictable.
What is Terrateam
Terrateam automates Terraform workflows using GitOps. When you open a pull request, it runs terraform plan and posts the results for review. Once approved and merged, it runs terraform apply, ensuring changes are reviewed and applied consistently across environments.
How to use Terraform and Terrateam to manage Flagsmith flags
Imagine you're rolling out a redesigned homepage for a React SPA with a Node.js backend. Instead of enabling it for everyone at once, the product team wants a gradual rollout. Flags need to stay consistent across Dev, QA, and Production, with QA always having the new UI for testing. If something breaks in Production, rolling back should be quick and predictable. With multiple teams involved, flag updates need to be version-controlled, reviewed, and applied consistently.
Step 1: Install Terrateam
- Sign up for Terrateam
- Install the Terrateam GitHub App by selecting the organisation and repository containing your Terraform feature flag code.
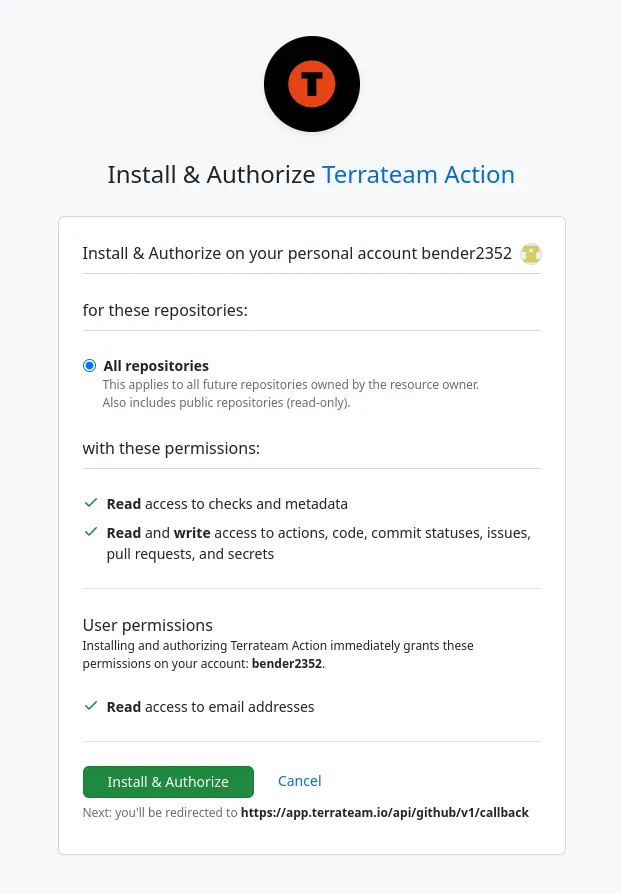
- Commit and push the .github/workflows/terrateam.yml to your default branch. This file is used to execute Terraform jobs using GitHub Actions.
- Grant Terrateam permission to access your cloud provider. This allows Terrateam to securely store Terraform state files with your existing cloud provider (configured in a later step). See Cloud Provider Setup for details.
- Create a .terrateam/config.yml file to configure how Terrateam handles Flagsmith pull requests.
Step 2: Add Flagsmith master API key to GitHub secrets
- In the Flagsmith dashboard, go to Organisation Settings > API Keys > Create API Key and copy your new key
- In GitHub, go to your repository's Settings > Secrets and Variables > Actions.
- Click New repository secret.
- Name the secret FLAGSMITH_MASTER_API_KEY and paste the key into the Value field.
- Click Add secret.
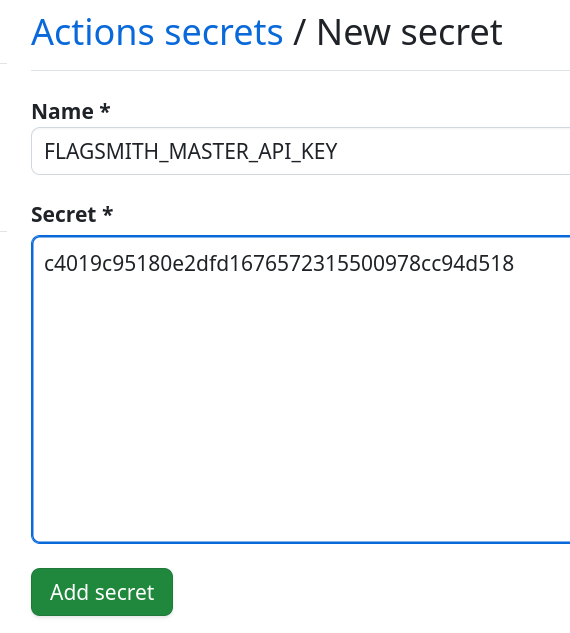
Step 3: Define feature flags in Terraform
Create a new feature branch in your repository and add a main.tf file to define your Flagsmith feature flag. Refer to the Flagsmith Terraform provider documentation for details on its capabilities.
Step 4: Open a pull request
Create a pull request with your new feature flag definitions. When the pull request is opened, Terrateam will detect the changes and initiate a Terraform plan. This allows you to review how the feature flag configuration will be applied before merging.
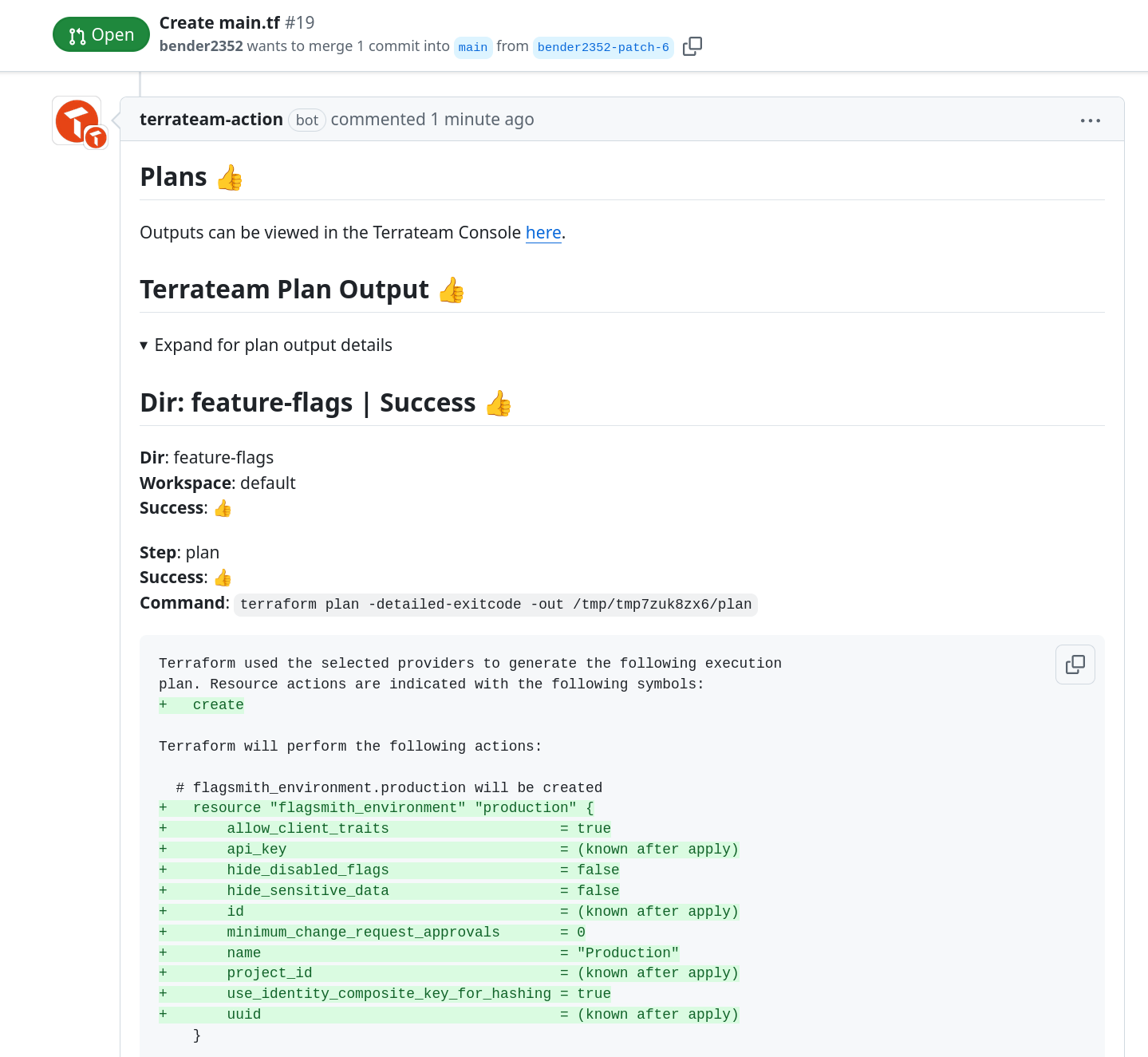
Once you're ready, merge the pull request, and Terrateam will automatically apply the changes using terraform apply.
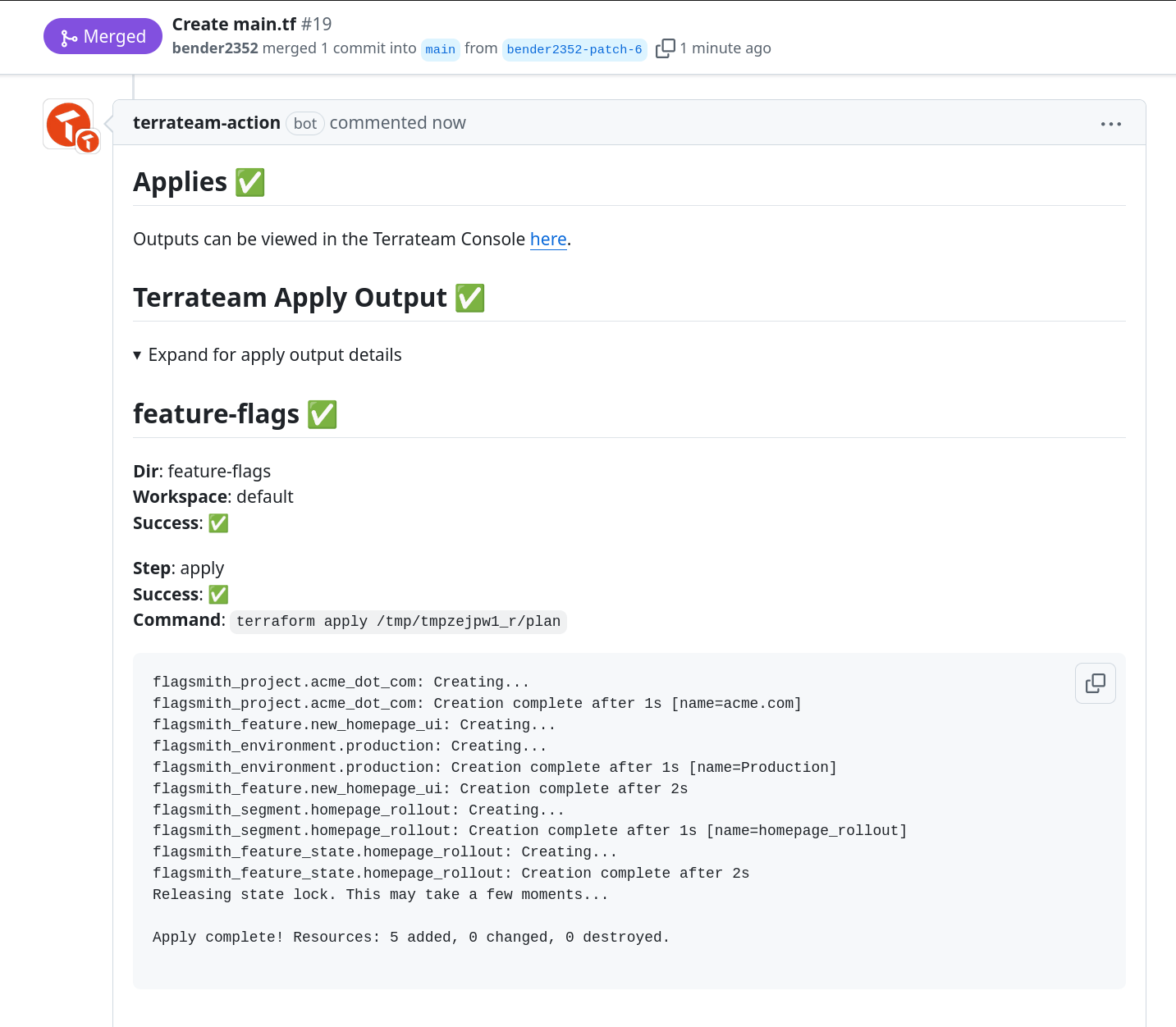
Step 5: Roll out the feature to Production
When the product team decides to roll out the new homepage UI to 10% of users in production, you update your Terraform configuration:
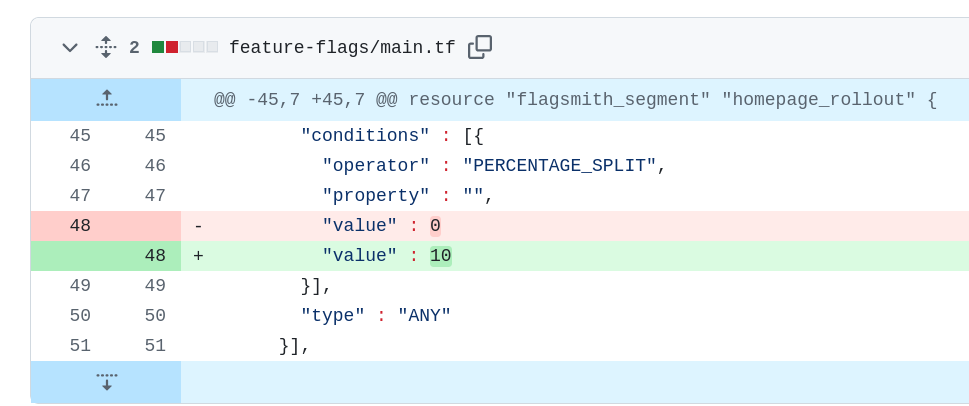
Open a pull request with this change, and Terrateam will generate a Terraform plan showing the proposed changes. Once the pull request is approved and merged, Terrateam applies the Terraform changes. The flag is updated in Flagsmith, and the rollout logic in your frontend automatically reflects the change. No manual toggling required.

Step 6: Instant rollback
To speed up rollbacks, you can pre-create a rollback PR that reverts the feature flag update. This can even stay in draft mode, ready to be merged instantly if needed. If an issue pops up, merging the rollback PR automatically sets the new_homepage_ui_mv_option back to 0. This makes rollbacks predictable as everything is stored in Git.
Use cases
Environment management
Different environments like development, staging, and production often require unique flag configurations. Managing these settings in code helps maintain consistency and reduces configuration drift. When environments need different settings, those differences are tracked in version control, so you always know why.
Canary deployments and feature deprecation
With canary deployments, you can deploy a feature to production while keeping it turned off for most users—letting you test it safely before rolling it out. As you begin to test with a small group of users before expanding your test groups, you can compare performance and optimise as you go. By managing these flag changes in Git, every rollout is tracked, reviewed, and version-controlled alongside your application code. When shutting down a feature, tracking its removal in Git makes it easy to audit and communicate to the team.
Some benefits
Using GitOps for feature flags keeps changes tracked, makes rollbacks easier, and keeps teams on the same page. For Flagsmith users, tools like Terrateam make managing flag configurations as natural as managing application code. It’s a practical way to reduce deployment risk and keep workflows smooth.
Integrating Flagsmith with GitOps tracks every flag change in version control, providing a clear audit trail. This keeps feature flags within the existing workflow, reducing operational overhead.
Including flag changes in code reviews allows engineers to evaluate updates as part of their normal process. If an issue comes up, rolling back means reverting a pull request. Applying the same review and CI/CD practices to feature flags helps maintain consistency across deployments.
Check out my conversation on Flagsmith’s The Craft of Open Source to hear more about Terrateam.
.webp)